CSS Basics and Styles
CSS works by pointing to (or selecting) HTML elements and modifying their presentation. For example, here's a simple semantic HTML document. (Remember this term from Lesson 3? Semantic HTML uses tags that explain what the different parts of the document mean rather than how they should look.)
If you save this document in a file with the extension .html and then open it in a Web browser, you'll see something like this:
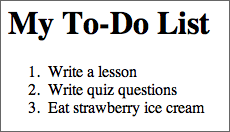
Simple HTML document
with no CSS applied
This webpage is rather plain. It's got some style, however. The header text is larger than the body text, there's space between the header and the body, the list has a numbered format, and the whole thing is in the Times New Roman font.
If you have an HTML document that doesn't contain any CSS, you'll see the browser default styles. Examples of browser default styles include the size of Heading 1 elements, the amount of line spacing between paragraphs, and the default text color and link color. All browsers use pretty much the same default style. These styles are never very attractive or exciting, however.
You don't want anyone to look at your app and think, "That looks boring." So here's an example of some CSS code that we could apply to this HTML to make it a bit more exciting.
And here's the result of applying these styles to the HTML document:
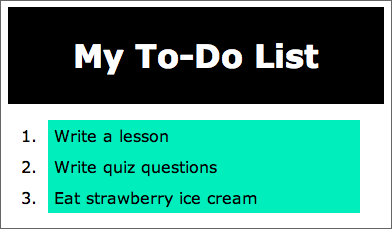
Document with CSS styles
I know, I know . . . it's still ugly. But at least it's a little more interesting!
Now that you've seen what I refer to as Chris' Simple Sample of CSS, let's discuss about how you can use CSS to beautify your own webpages.
Adding CSS to HTML Documents
You have three choices for how to add CSS to HTML documents:
- With inline styling, you add CSS to individual elements within a style attribute.
- Using page-level styles lets you add CSS to an entire page using the <style> element.
- An external style sheet uses the <link> element to connect to a separate CSS document.
To find out how to use each of these methods, and to start understanding the power of style sheets, let's take a quick look at the WatzThis.com documentation we created in Lesson 3.
Let's say you wanted to change the typeface of all the text in this document from the default serif font to a sans-serif font.
Sans What?
When I say serif, I'm talking about the little lines or rounded edges on the ends of letters. Some typefaces have these little lines and edges; typefaces that don't are sans-serif. ("Sans" is French for "without.")
Serif typeface Sans-serif typeface
Designers and typographers love to argue about which type of font is better. Some people say a sans-serif typeface gives text a cleaner look. Others point to studies indicating that serif typefaces are easier to read.
As you build your own apps, experiment with different typefaces. Which ones work best to get your message across?
Using inline styling, you'd simply add a style attribute to every element in the body that contains text. Here's how that would look (I've highlighted the changes):
And here's what the document looks like when you view it in a browser:
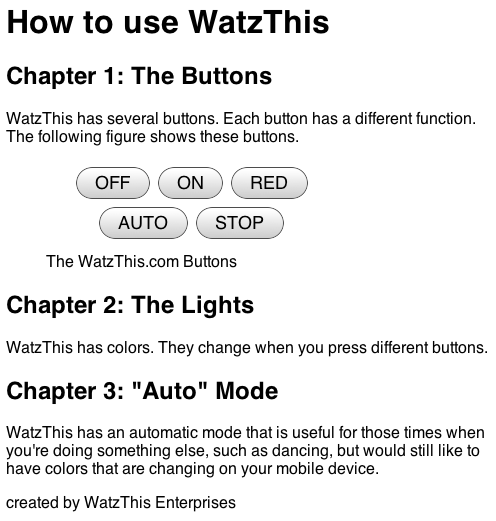
Using CSS rules to change the type to a sans-serif font
Like most structured documents, the WatzThis documentation contains repeated elements. For example, this document contains three <section> elements, three <h2> elements, and three <p> elements. The marketing department has mentioned that they don't consider this the final document yet (I never would have guessed!), so there may be many more of each of these elements in the future.
It sure looks better with that sans-serif font . . . but you've added a considerable amount of text to the HTML file, which has made the file more difficult to edit and increased the file size (and therefore has made it take longer to download).
When you need to style just one element in just one document, inline styling is an acceptable technique. More often, however, you'll need to apply a style to multiple elements within a document. This is where page-level styling comes in.
Here's how you can add a new font family to the text in this document using page-level styling. Again, I've highlighted the new code.
As you can see, this document looks the same in a Web browser.
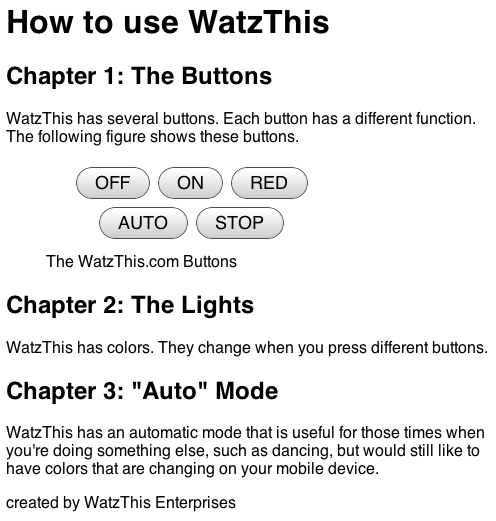
Documentation with our new, optimized CSS
Page-level styling lets you use one style rule to change everything that matches that rule in the document.
As far as the result is concerned, the only difference between the document with inline styles and the one with page-level styles is that one of them is 1,086 characters long and the other is 851. This means the second document will take about 20% less time to download.
Page-level CSS styles are also much easier to maintain than inline styles. Imagine that the marketing manager comes back to you the next day and asks you to change the font back to serif or to put it all in Comic Sans. With inline styles, you'll need to carefully go through the document and change the font-family property's value in each place it occurs. With page-level CSS, you need to make only one change to affect the whole document.
But what if the marketing manager comes back again? This time she says to split each section of documentation into a separate page, and, oh yeah, management has requested that the biographical information and photos of the company management also appear on a separate page. They'd also like to have a "contact us" page that lists a technical support phone number and email address (yours, of course) that people can use if they need help.
Now you've got at least five HTML pages to style. If you use page-level CSS rules, you're suddenly faced with five things you need to change when the corporate font changes. When you have multiple pages, you should use an external style sheet.
External style sheets are files with a .css extension that contain style rules that you want to apply to multiple files. Here's an external style sheet with the style rule that we've created for the WatzThis documentation:
Copy this code. Now create a new blank document in Komodo Edit and paste it in there. This should be the only text in the file.
Save the file into the same directory as the HTML file that you want to style. Make sure to save the file with a .css extension. Then, to use it in as many HTML documents as you want, just link to it with this code inside the <head> element:
Naturally, you'd use the name of the CSS file you created rather than watzthis.css.
I asked you to save the CSS file into the same directory as your HTML file for simplicity's sake. It's actually more common to save CSS files in their own directory, separate from HTML files. If you were to save this CSS file in a directory called 'css', your <link> element would look like this:
Once you've included the external style sheet in every page of your site, all you need to do to change the font sitewide is to modify the style in this one file. Pretty cool, huh?
About User Agents
Ease of maintenance is just one benefit of using style sheets. Another is flexibility in where and how a browser presents your document.
A program that uses HTML content in any way is a user agent. Web browsers are one type of user agent; mobile browsers are another. Email programs commonly read HTML content and can be user agents too. Other types of user agents include search engine spiders, Braille browsers, and screen readers.
CSS lets you have one HTML document that you can style in multiple ways to fit multiple types of user agents. Later in this lesson, I'll show you one way to style documents differently for different users.
By using CSS, you can create different style rules for how different user agents should use a single HTML document. One of the main objectives of this course is to show you how to style the same content for desktop browsers and mobile devices. This wouldn't be possible without CSS.
Now that you understand the importance of CSS, let's dig deeper into the language and find out how it works.