Using Web APIs
Web Application Programming Interfaces allow developers to make parts of their apps' functionality or data available to other apps. I know what you're thinking: Why would someone who has invested time and money developing an app want to share their data and functionality with others for free?
Welcome to Web App Marketing 101, my friend. A Web business might allow outside apps access to its data or functionality for many reasons.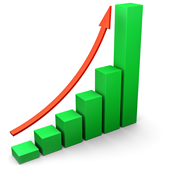
- Exposure. Millions of Web businesses compete for the eyeballs of Web surfers. For most of these businesses, it's a struggle to acquire new customers . . . or to acquire them faster and keep them longer than the competition. A Web API that other businesses find valuable enough to use puts your message in front of more users.
- Functionality. If your app has a Web API that makes it easy to integrate with other Web apps, potential customers will see this as another reason to choose you over the competition.
- Advertising. Providing free data to users in exchange for users viewing messages from advertisers is the oldest business model on the Web. Publishing a Web API is one way to reach a larger audience.
Now that you have a better idea why a business would create a Web API, let's look at the building blocks of Web APIs. After that, we'll take a look at some examples of APIs and how you can use them.
REST, XML, and JSON: Three Keys to Creating Interfaces
A great number of Web APIs rely on three simple technologies: REST, XML, and JSON.
REST stands for Representational State Transfer. It's a flexible way to expose functionality of a Web app by using meaningful URLs. The term Representational State Transfer is a fancy way of saying, "Use ordinary Web links that represent what you're asking for to get what you want."
A Web API that uses REST is a RESTful Web API.
For example, Geonames.org provides a variety of Web APIs. One of these tells you thecurrent time based on your latitude and longitude. To use this API, you simply access it usingthe following format (where the ?? represents variables).
http://api.geonames.org/timezone?lat=??&lng=??&username=??
If you find out your current location (using the modification we made to WatzThis in Lesson7, for example), you can find your current time zone by plugging the latitude and longitudeinto the appropriate places in the URL. For example:
http://api.geonames.org/timezone?lat=47.01&lng=10.2&username=demo
If you type or paste this URL into a browser, the Geonames.org Web API will return some data.
A RESTful Web API usually returns data in an XML or JSON format. We'll get to JSON in aminute; let me give you the details on XML first.
XML (Extensible Markup Language) is a markup language, like HTML, that's easy to read. Ithas stricter syntax rules than HTML, however, which also makes it useful forcommunications between computers, where having precise data matters.
The main syntax differences between XML and HTML are:
- XML is case sensitive. As you learned in Lesson 6, most computer languages are casesensitive. In other words, they don't recognize "T" and "t" as being the same.
- Every XML element must be closed. Unlike HTML, XML doesn't permit openingtags without matching closing tags or a closing slash in an empty element.
Here's a sample of XML data that you get when you use the Geonames.org URL listed above:
Notice that the basic structure of the document resembles HTML in that it uses tags to create elements that describe data.
However, here's the important difference between XML and HTML: XML has no predefined elements. As long as you stick to the syntax rules and some additional restrictions on which characters are valid (no spaces in element names, for example), you can use pretty much anything as an element name. So the following are all perfectly okay XML elements:
<taco></taco>
<salsa></salsa>
<cerveza></cerveza>
You can put content between the beginning and ending tags, just as you would in HTML, and the tags provide more information about the purpose of the content.
Extensible Markup Language means that you can "extend" the language by creating your own tags. You don't have to wait for some other person or organization to invent and approve the tags you need right now.
Take the example of the <taco> element I created earlier. If you wanted to describe your taco, here's one way you might do it with XML:
Notice that this markup code is readable to you and me, and that it provides almost all the information that you need to create the perfect vegetarian taco, along with the proper nesting of elements (the filling goes inside the tortilla, for example). None of the elements are predefined—I just made them up as I needed them.
Even though you're free to create your own XML elements as you wish (and this is exactly what most people do when working with Web APIs), there are sets of predefined XML elements, called vocabularies, which experts have created for different purposes. For example, a standardized XML recipe vocabulary might specify the elements you should use to write a recipe.
In the next lesson, we'll look at one popular XML vocabulary, SVG, for creating graphics on the Web.
JSON (JavaScript Object Notation) is a newer and lighter-weight data-exchange language than XML. It's becoming increasingly popular for Web apps because it's easy to use inside JavaScript code. Why is it so easy to use? Because it actually is JavaScript code.
JSON packages data as JavaScript objects with the following format:
Here's what the XML example above looks like when you convert it to JSON:
Notice that the JSON representation of this data uses less than half of the number ofcharacters (and therefore less storage space).
Once JSON data is inside a running JavaScript program, you can access the data inside it using dot notation. For example, here's how you can access different parts of the above geonames data:
Many Web APIs provide their output data in XML and JSON formats. The New York Times is a great example. Once you sign up as a developer at developer.nytimes.com, you can access articles, best sellers, event listings, movie reviews, and more by using their RESTful Web APIs.
For example, the following URL selects movie reviews using the keyword "airplane":
http://api.nytimes.com/svc/movies/v2/reviews/search.json?query=airplane&api-key={your-API-key}
To retrieve the same data as XML, just change the extension after "search":
http://api.nytimes.com/svc/movies/v2/reviews/search.xml?query=airplane&api-key={your-API-key}
Why don't these URLs work? Notice that each one contains a parameter called "api-key." Many Web APIs require you to register as a developer and obtain a unique ID, called an API key, to access their service.
Including the API key helps the app owners make sure that you've agreed with their terms of service and gives the provider of the Web service some information about who's using their API. In most cases, requesting the API key is a simple process and takes only a few minutes, as you'll see in this lesson's assignment.
Here's a sample of the data that the above request returns (assuming that you provide a valid API key):
Just as you can click a link or paste the URL for a RESTful Web API into a browser address bar, Web apps that programmers write can request data through the same URL. Then, when the Web app on the other end responds with XML or JSON code, the Web app can convert the response into a useful format and do things with it (such as show it to the user or use it in its own calculations).
Now that you know a little bit about Web APIs, let's explore the answer to this question: How do people combine their data (or functionality, or both) to make mashups? Please move ahead to Chapter 3.